The PHP array can be used in the javascript function in many ways. But today in this blog we will use the main two ways. The first one is json_encode() and the second one is most common PHP implode() function.
Method 1 : json_encode()
First, define an array into PHP. json_encode() returns the JSON representation of a value.
<?php
$phpArray = array(
0 => "Zero",
1 => "One",
2 => "Two",
3 => "Three",
4 => "Four",
5 => "Five",
6 => "Six",
7 => "Seven",
8 => "Eight",
9 => "Nine",
10 => "Ten"
)
?>
Now we use PHP array into javascript. Retrieve the array elements using json_encode() and use a loop to print js value.
<script type="text/javascript">
var jsArray = <?php echo json_encode($phpArray); ?>;
for(var i=0; i<jsArray.length; i++){
console.log(jsArray[i]);
}
</script>
Full code
<?php
// Create an array in PHP
$phpArray = array(
0 => "Zero",
1 => "One",
2 => "Two",
3 => "Three",
4 => "Four",
5 => "Five",
6 => "Six",
7 => "Seven",
8 => "Eight",
9 => "Nine",
10 => "Ten"
)
?>
<script type="text/javascript">
// Retrieve the PHP array elements using json_encode() into jascript
var jsArray = <?php echo json_encode($phpArray); ?>;
// Run a loop to print array value
for(var i=0; i<jsArray.length; i++){
console.log(jsArray[i]);
}
</script>
Method 2: implode()
In the second method, we use another PHP method implode(). It is used to join array elements with a string. implode() is also known as the PHP join() function and it works similar to that of the join() function.
<?php
// Create an array in PHP
$phpArray = array(
0 => "Zero",
1 => "One",
2 => "Two",
3 => "Three",
4 => "Four",
5 => "Five",
6 => "Six",
7 => "Seven",
8 => "Eight",
9 => "Nine",
10 => "Ten"
)
?>
<script type="text/javascript">
// Using PHP implode() function
var jsArray = <?php echo '["' . implode('", "', $phpArray) . '"]' ?>;
for(var i=0; i<jsArray.length; i++){
console.log(jsArray[i]);
}
</script>
Result

By both the method you will get the same result. I hope this post is helpful for you.
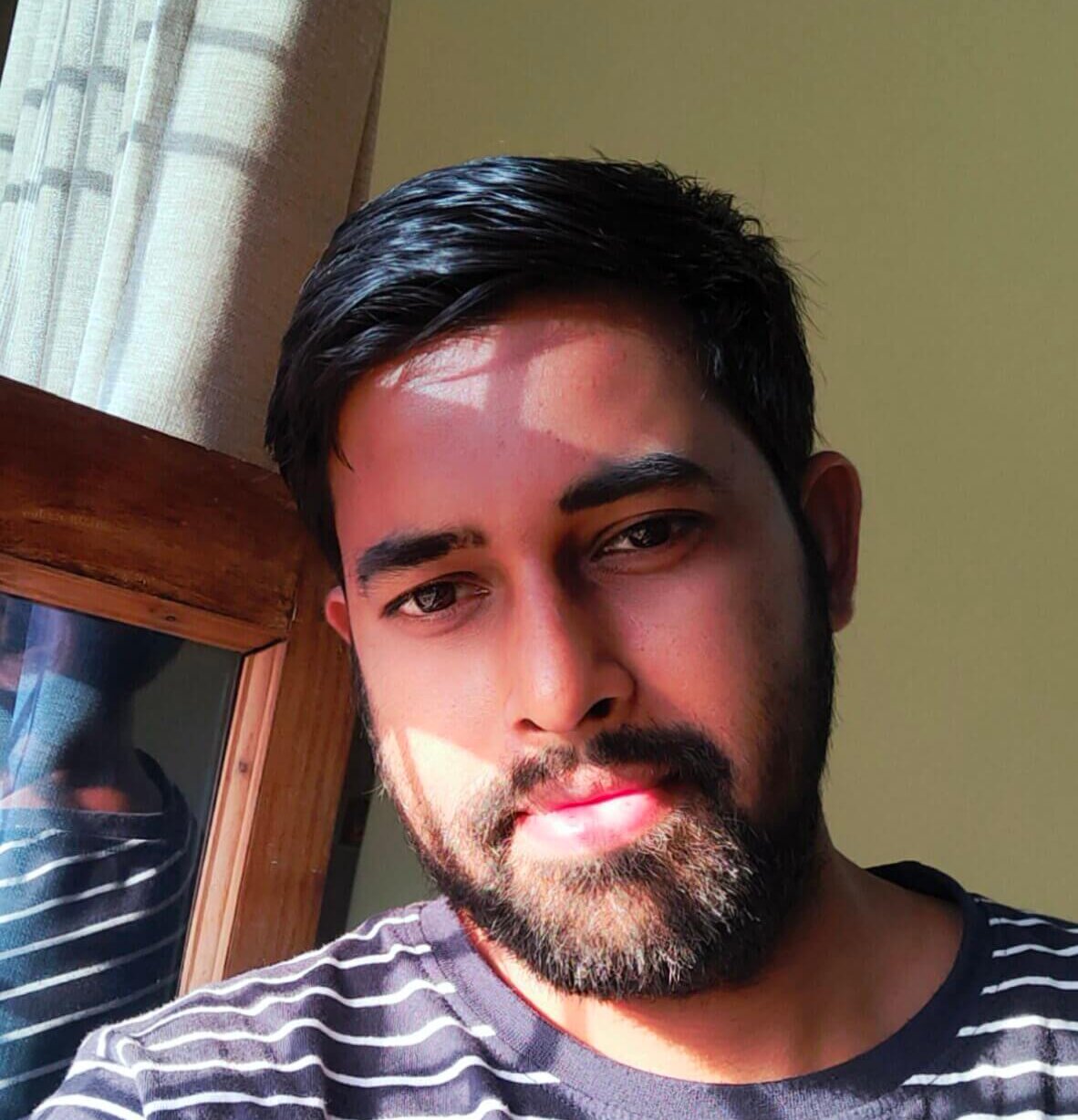
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.