In this blog post, we learn How To Make Multi Auth In Laravel 8. We know laravel gives us a default auth for users but in some cases, we need another auth for admin or seller.
So first here I am using a fresh laravel 8 application so first, install a laravel application and generate user auth and create a new admin auth.
Step: 1 Create a New Laravel 8 Application
create a new Laravel 8 Application using this command.
laravel new multiauth
After successfully create a project run some commands to generate default auth.
composer require laravel/ui --dev
and
php artisan ui bootstrap --auth
after that run the following command
npm install && npm run dev
After running the above command you may get this message.

So this command
npm run dev
Now you have some pages such as login, register, and forget the password.
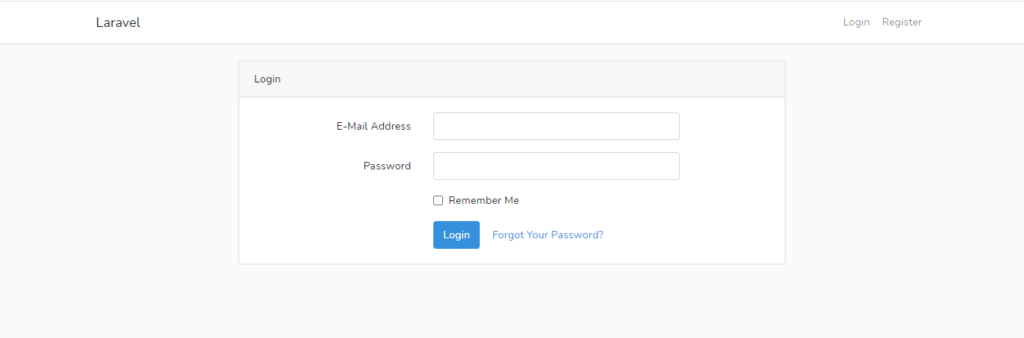
Ok, We completed user auth but we need to create another auth for admin. So we need another table for admin detail. Therefore create a new model and migration file.
Step: 2 Create a Model and Migration File for Admin
Now we are creating an Admin model and migration file for admin using the flowing command
php artisan make:model Admin -m
Add some code in the admin model
Models / Admin.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Foundation\Auth\User as Authenticatable;
class Admin extends Authenticatable
{
use HasFactory;
protected $guard = 'admin';
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name', 'email', 'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password', 'remember_token',
];
}
Admin some code for the admin migration file. You can add more according to your project.
public function up()
{
Schema::create('admins', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->timestamps();
});
}
Update your .env file to connect the database and run this command to migrate the user table.
php artisan migrate
Now we need to modify auth.php and add some custom guards and providers for admins.
config / auth.php
'guards' => [
'web' => [
'driver' => 'session',
'provider' => 'users',
],
'api' => [
'driver' => 'token',
'provider' => 'users',
],
'admin' => [
'driver' => 'session',
'provider' => 'admins',
],
'admin-api' => [
'driver' => 'token',
'provider' => 'admins',
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\User::class,
],
'admins' => [
'driver' => 'eloquent',
'model' => App\Models\Admin::class,
],
],
Step: 3 Create Admin Login Page and Admin Dashboard Page
auth / admin_login.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=UTF-8>
<meta name=viewport content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Admin</title>
</head>
<body>
<div class="container">
<div class="row justify-content-center mt-4">
<div class="col-md-4">
<div class="card">
<div class="card-body">
<form id="sign_in_adm" method="POST" action="{{ route('admin.login.submit') }}">
{{ csrf_field() }}
<h1>Admin Login</h1>
<div >
<input type="email" name=email class="form-control" placeholder="Email Address" value="{{ old('email') }}" required autofocus>
</div>
@if ($errors->has('email'))
<span ><strong>{{ $errors->first('email') }}</strong></span>
@endif
<br>
<div >
<input type="password" name="password" class="form-control" required>
</div>
<br>
<div >
<button type="submit" class="btn btn-primary">Admin Login</button>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Result

admin.blade.php create admin dashboard page when admin redirect after login
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset=UTF-8>
<meta name=viewport content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Admin Dashboard</title>
</head>
<body>
<nav class="navbar navbar-light bg-light">
<div class="container-fluid">
<a class="navbar-brand">Admin Dashboard</a>
<a href="/admin/logout">Logout</a>
</div>
</nav>
<div class="alert alert-success" role="alert">
Welcome to admin dashboard
</div>
</body>
</html>
Step: 4 Create AdminController and AdminLoginController
Run this command to create an AdminController in the auth folder.
php artisan make:controller Auth/AdminController
Add some code in AdminController.php
<?php
namespace App\Http\Controllers\Auth;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
class AdminController extends Controller
{
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth:admin');
}
/**
* show dashboard.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('admin');
}
}
Run this command to create an admin login Controller.
php artisan make:controller Auth/AdminLoginController
AdminLoginController.php
<?php
namespace App\Http\Controllers\Auth;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use Auth;
use Route;
class AdminLoginController extends Controller
{
public function __construct()
{
$this->middleware('guest:admin', ['except' => ['logout']]);
}
public function showLoginForm()
{
return view('auth.admin_login');
}
public function login(Request $request)
{
// Validate the form data
$this->validate($request, [
'email' => 'required|email',
'password' => 'required|min:6'
]);
// Attempt to log the user in
if (Auth::guard('admin')->attempt(['email' => $request->email, 'password' => $request->password])) {
// if successful, then redirect to their intended location
return redirect()->intended(route('admin.dashboard'));
}
// if unsuccessful, then redirect back to the login with the form data
return redirect()->back()->withInput($request->only('email', 'remember'));
}
public function logout()
{
Auth::guard('admin')->logout();
return redirect('/admin');
}
}
Step: 5 Create some routes for admin.
Add some routes for handle admin.
Route::prefix('admin')->group(function() {
Route::get('/login','Auth\AdminLoginController@showLoginForm')->name('admin.login');
Route::post('/login', 'Auth\AdminLoginController@login')->name('admin.login.submit');
Route::get('logout/', 'Auth\AdminLoginController@logout')->name('admin.logout');
Route::get('/', 'Auth\AdminController@index')->name('admin.dashboard');
}) ;
Step: 6 Handle admin redirection to login page or dashboard
After that, we need to add some code to handle redirection for login and logout.
Add some function in app/Exceptions/Handler.php
<?php
namespace App\Exceptions;
use Illuminate\Auth\AuthenticationException;
use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler;
use Illuminate\Support\Arr;
use Throwable;
class Handler extends ExceptionHandler
{
/**
* A list of the exception types that are not reported.
*
* @var array
*/
protected $dontReport = [
//
];
public function report(Throwable $exception)
{
parent::report($exception);
}
public function render($request, Throwable $exception)
{
return parent::render($request, $exception);
}
protected function unauthenticated($request, AuthenticationException $exception)
{
if ($request->expectsJson()) {
return response()->json(['error' => 'Unauthenticated.'], 401);
}
$guard = Arr::get($exception->guards(), 0);
switch ($guard) {
case 'admin':
$login='admin.login';
break;
default:
$login='login';
break;
}
return redirect()->guest(route($login));
}
/**
* A list of the inputs that are never flashed for validation exceptions.
*
* @var array
*/
protected $dontFlash = [
'current_password',
'password',
'password_confirmation',
];
/**
* Register the exception handling callbacks for the application.
*
* @return void
*/
public function register()
{
$this->reportable(function (Throwable $e) {
//
});
}
}
After that, we need to change auth redirection when the admin login it should be redirected to the right place such as the admin dashboard. update the following code in redirect if authenticated.php
app\Http\Middleware\RedirectIfAuthenticated.php
<?php
namespace App\Http\Middleware;
use App\Providers\RouteServiceProvider;
use Closure;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class RedirectIfAuthenticated
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @param string|null ...$guards
* @return mixed
*/
public function handle($request, Closure $next, $guard = null)
{
switch ($guard) {
case 'admin':
if (Auth::guard($guard)->check()) {
return redirect()->route('admin.dashboard');
}
default:
if (Auth::guard($guard)->check()) {
return redirect('/');
}
break;
}
return $next($request);
}
}
Ok, here we completed tutorials on How To Make Multi Auth In Laravel 8. run your application using this flowing command.
php artisan serve
May you got this error in Laravel
Target class [Auth\AdminController] does not exist
Define namespace in RouteServiceProvider as an old version.
Read this post Target class Controller does not exist
App\Providers\RouteServiceProvider
public function boot()
{
$this->configureRateLimiting();
$this->routes(function () {
Route::prefix('api')
->middleware('api')
->namespace($this->namespace)
->namespace('App\Http\Controllers') <------------ Add this
->group(base_path('routes/api.php'));
Route::middleware('web')
->namespace($this->namespace)
->namespace('App\Http\Controllers') <------------- Add this
->group(base_path('routes/web.php'));
});
}
and visit this URL to log in to the admin login page. //localhost:8000/admin

I hope this post will be of use to you.
You will get the full code of the Make Multi Auth In Laravel 8 project in my GitHub account, you can take help from here.
Full working source Code of multi auth in laravel 8.
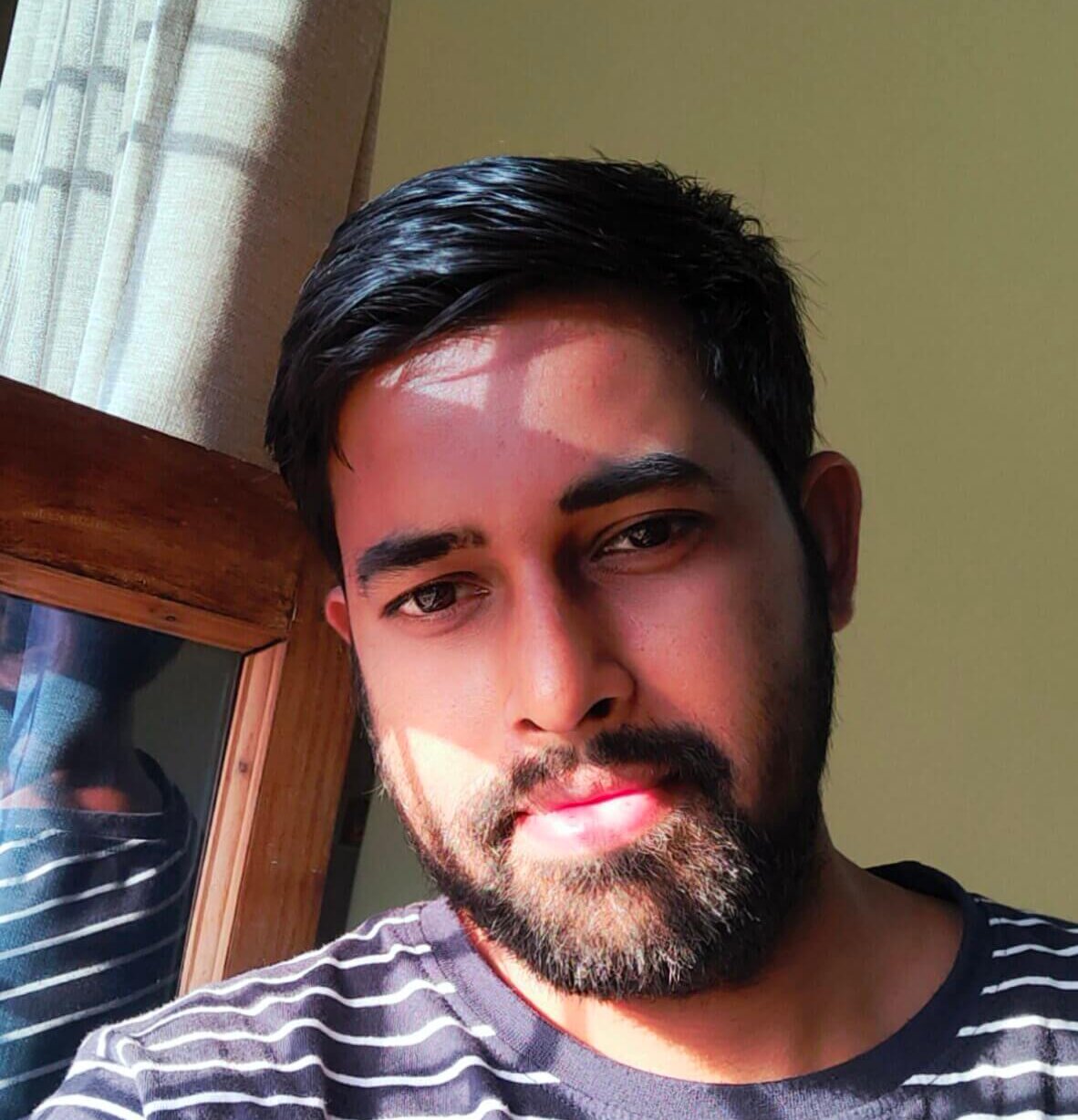
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.