To match patterns over multiple lines in Python, you can use the re
module along with the re.DOTALL
or re.S
flag. These flags allow the dot (.
) metacharacter to match any character, including newlines. Here’s an example:
import re
# Multiline string
text = '''
This is line 1.
This is line 2.
This is line 3.
'''
# Regular expression pattern with dotall flag
pattern = r'This is line \d\.'
# Match pattern over multiple lines
matches = re.findall(pattern, text, re.DOTALL)
# Print the matches
print(matches)
In this example, the pattern This is line \d\.
matches lines that start with “This is line ” followed by a digit and a dot. The re.DOTALL
flag is passed as the third argument to re.findall()
, enabling the matching of patterns across multiple lines.
The output of the above code will be:
['This is line 1.', 'This is line 2.', 'This is line 3.']
Alternatively, you can use the shorthand flag re.S
instead of re.DOTALL
. Both flags have the same effect of enabling multiline matching with the dot metacharacter.
By using these flags, you can match patterns that span across multiple lines in Python regular expressions.
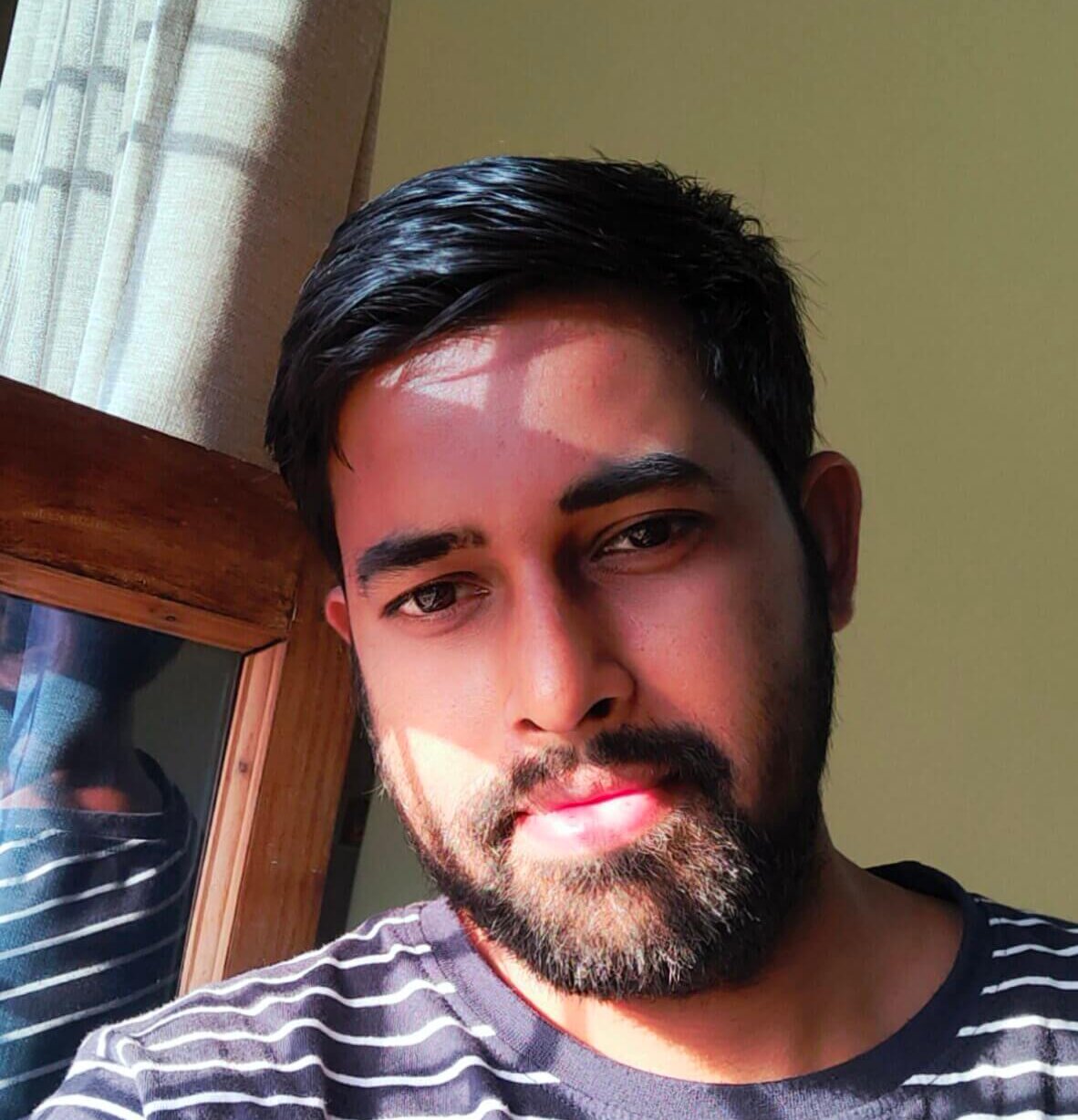
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.