In JavaScript, both the substring()
and slice()
methods are used to extract portions of a string. While they have similar functionality, there are a few differences between them:
- Parameter Handling: The
substring()
method takes two parameters: the starting index and the ending index. If the starting index is greater than the ending index, the method will swap the values internally to ensure correct extraction. On the other hand, theslice()
method also takes two parameters, but it allows negative values. Negative values are counted from the end of the string. For example, a negative index of -1 represents the last character in the string. - Mutability of Original String: The
substring()
method does not modify the original string; it returns a new substring. In contrast, theslice()
method can modify the original string in certain cases. If theslice()
method is called on an array or a string object, it returns a new array or string object. However, if it is called directly on a string primitive, it can modify the string primitive itself. - Compatibility: The
substring()
method is part of the JavaScript specification and is supported by all major browsers. On the other hand, theslice()
method is also widely supported, but it was introduced in ECMAScript 3 and may not be available in older browsers.
Here are examples of using both methods to extract substrings:
var str = "Hello, World!";
var substring = str.substring(0, 5);
console.log(substring); // Output: Hello
var sliced = str.slice(7, 12);
console.log(sliced); // Output: World
var negativeSliced = str.slice(-6, -1);
console.log(negativeSliced); // Output: World
In the above code, substring()
and slice()
are used to extract different portions of the string str
. The substring()
method is used to extract the substring “Hello”, while the slice()
method is used to extract the substrings “World” and “World” using both positive and negative indexes.
In general, slice()
is more flexible due to its support for negative indexes, but substring()
is still widely used and can handle most substring extraction scenarios.
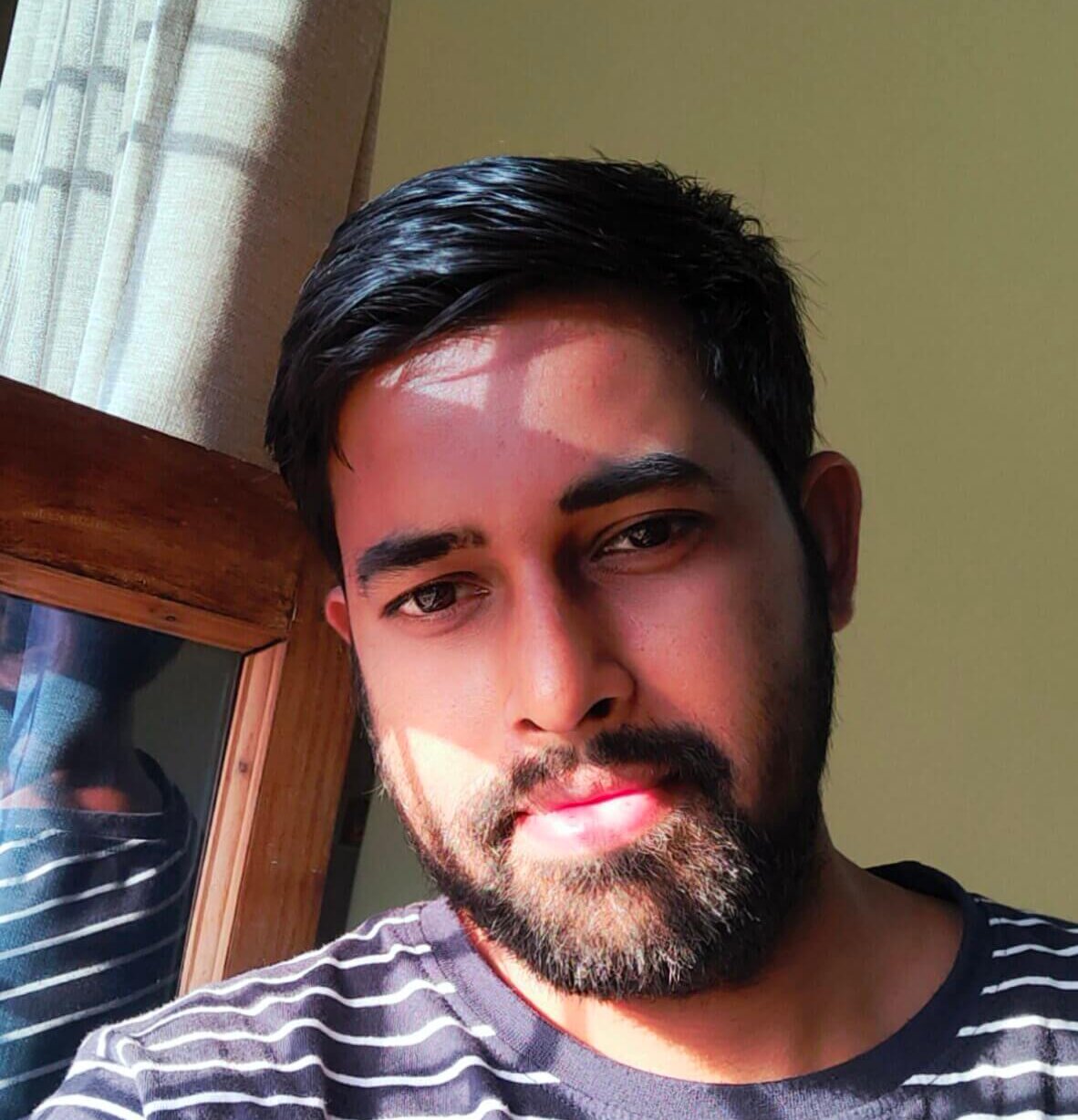
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.