In this post, I explain how to check Table/Column already exist or not in Laravel.
When we working on a big project generally we faced migration for the same table or even column that already exists. Luckily, Laravel has a quick way of checking it.
Typical migration file looks like this:
public function up()
{
Schema::create('flights', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('airline');
$table->timestamps();
});
}
ok, Suppose if flights table already exists in our database, you would get an error. The solution to this is Schema::hasTable() method.
public function up()
{
if (!Schema::hasTable('flights')) {
Schema::create('flights', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('airline');
$table->timestamps();
});
}
}
By using this migration method, if table flights do not exist, then we create it.
if we want to add a new column to the existing table, then we may check if the table does exist.
Let’s combine this with another example and with another method Schema::hasColumn(), which has two parameters – table name and column name.
public function up()
{
if (Schema::hasTable('flights')) {
Schema::table('flights', function (Blueprint $table) {
if (!Schema::hasColumn('flights', 'departure_time')) {
$table->timestamp('departure_time');
}
});
}
}
You can read more about migrations and its various features in the official documentation.
So here we completed post on Laravel Migrations: Check if Table/Column Already Exists
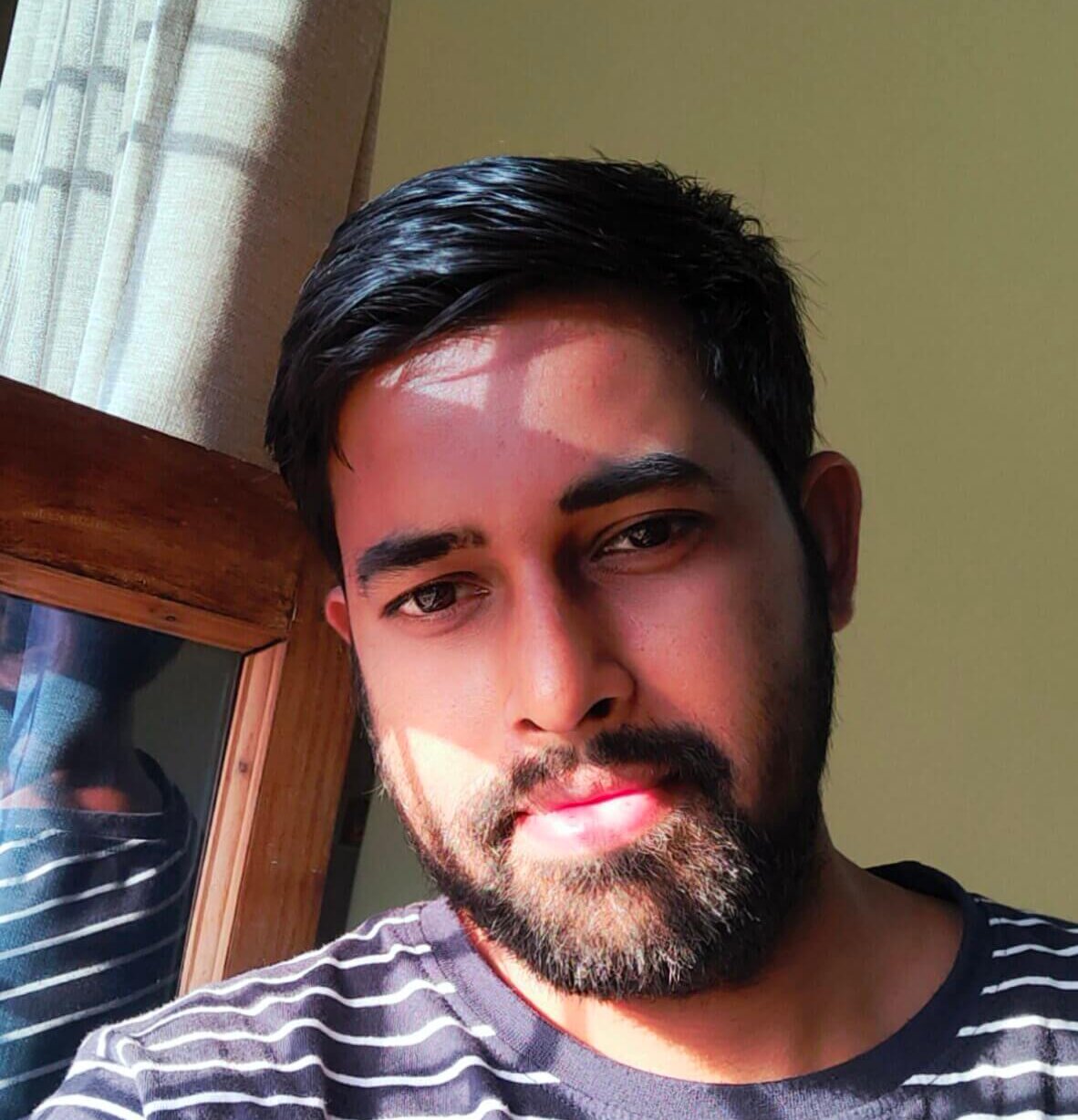
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.