To remove the last character from a string using JavaScript’s substring()
method, you can specify the desired range of characters to extract. Here’s an example:
var str = "Hello, World!";
var substring = str.substring(0, str.length - 1);
console.log(substring); // Output: Hello, World
In the above code, str.length - 1
is used as the endIndex
parameter for the substring()
method. This subtracts 1 from the length of the string, effectively excluding the last character from the extracted substring.
After removing the last character, the resulting substring will be assigned to the substring
variable. In this example, the output will be “Hello, World” without the exclamation mark.
Keep in mind that the substring()
method doesn’t modify the original string but instead returns a new string. If you need to remove the last character from the original string permanently, you can reassign the modified substring back to the original variable, like this:
str = str.substring(0, str.length - 1);
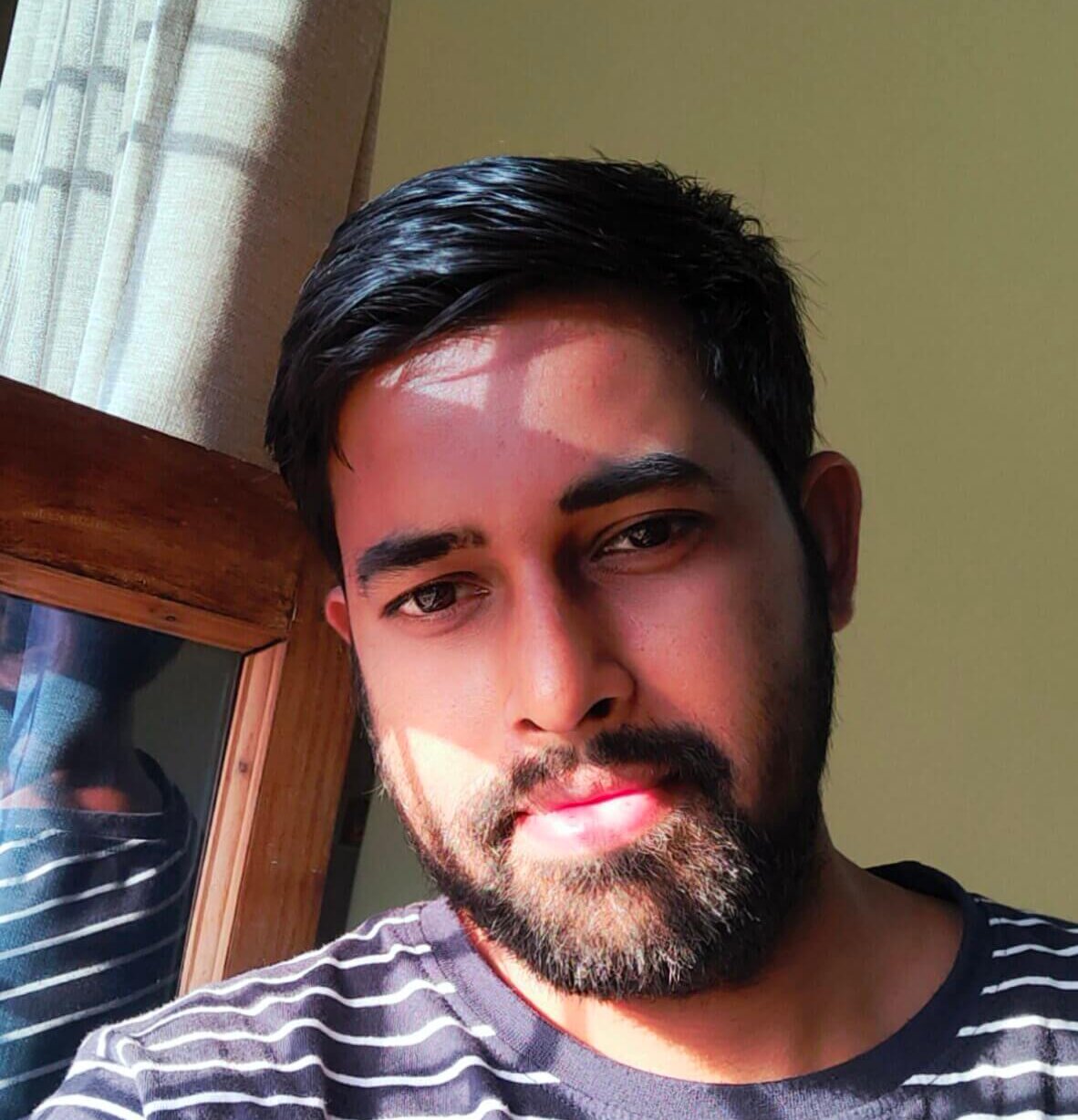
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.