Certainly! In Python, you can split strings using the split()
method or the re.split()
function from the re
module for more complex splitting based on regular expressions. Here are examples of both approaches:
Splitting with split()
method:
sentence = "Hello, how are you today?"
words = sentence.split() # Split by whitespace
print(words) # Output: ['Hello,', 'how', 'are', 'you', 'today?']
numbers = "1,2,3,4,5"
number_list = numbers.split(",") # Split by comma
print(number_list) # Output: ['1', '2', '3', '4', '5']
The split()
method without any arguments splits the string by whitespace by default. You can also provide a specific separator character or substring as an argument to split the string based on that separator.
Splitting with re.split()
function:
import re
sentence = "Hello, how are you today?"
words = re.split(r'\W+', sentence)
print(words) # Output: ['Hello', 'how', 'are', 'you', 'today']
numbers = "1,2,3,4,5"
number_list = re.split(r',', numbers)
print(number_list) # Output: ['1', '2', '3', '4', '5']
The re.split()
function splits the string based on a specified regex pattern. In the examples above, \W+
matches one or more non-word characters, including punctuation marks, to split the sentence into words. Similarly, the comma (,
) is used as the separator pattern to split the numbers string.
Note: When using re.split()
, be mindful of the regex pattern you provide. It allows for more advanced splitting scenarios, but it’s important to ensure the pattern matches your desired splitting criterion.
Both approaches allow you to split strings in Python based on specific criteria, whether it’s whitespace, a specific character, or a regex pattern. Choose the method that suits your requirements and manipulate the resulting list of substrings as needed.
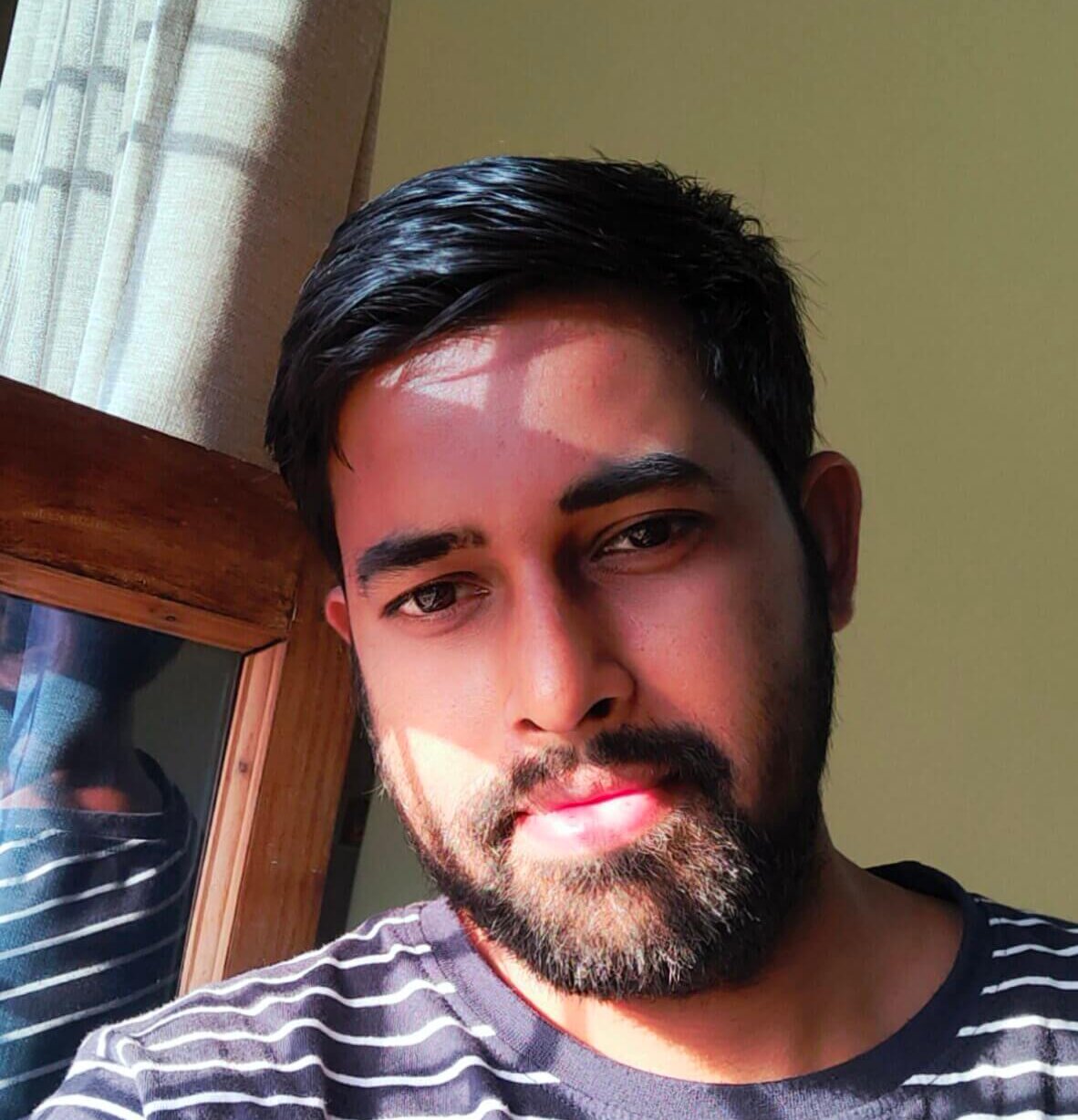
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.