In this blog, we learn How To Display A Loader While Sent Request Is In Progress In Vue.
So, To demonstrate this loader function. we will create a component for login or register and then send a request to the server. while sending a request to the server we will show a loader animation or image on the page of login. so let’s start.
Step: 1 Create a Login or Register component
Here I create a login component
Login.vue
<template>
<div >
<div v-if="loading">
<!-- here put a spinner or whatever you want to do when request is on proccess -->
<div ></div>
</div>
<div v-if="!loading" >
<div >
<div >
<div >Login vue</div>
<div >
<form>
<div >
<label for="email" >E-Mail Address</label>
<div >
<input id="email" type=email v-bind: v-model="email" required autofocus>
<small >{{ error }} </small>
</div>
</div>
<div >
<label for="password" >Password</label>
<div >
<input id="password" type=password v-model="password" required>
<router-link :to="{ name: 'email' }" >Forget Your Password ?</router-link>
</div>
</div>
<div >
<div >
<button type=submit @click="handleSubmit">
Login
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</template>
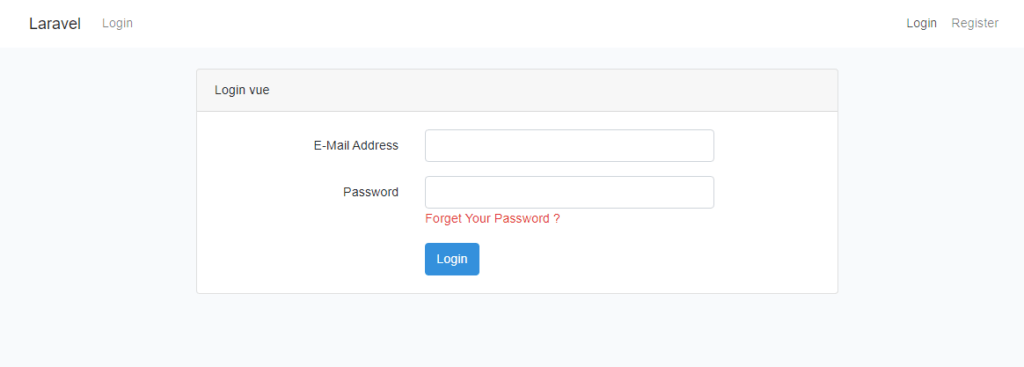
After that add CSS code for the Loader image.
<style type=text/css>
.js #loader { display: block; position: absolute; left: 100px; top: 0; }
.se-pre-con {
position: fixed;
left: 0px;
top: 0px;
width: 100%;
height: 100%;
z-index: 99;
background: url("//wpamelia.com/wp-content/uploads/2018/11/ezgif-2-6d0b072c3d3f.gif") center no-repeat #fff;
}
</style>
Add Vue Code
<script>
export default {
data(){
return {
email : "",
password : "",
error : "",
loading: false,
name: 'vue-title',
props: ['title'],
}
},
methods : {
handleSubmit(e) {
e.preventDefault()
if (this.password.length > 0){
this.loading = true ;
axios.post('api/login', {
email: this.email,
password: this.password
}).then((response) => {
let is_admin = response.data.user.is_admin
localStorage.setItem('user',JSON.stringify(response.data.user))
localStorage.setItem('jwt',response.data.token)
this.loading = false;
if (localStorage.getItem('jwt') != null){
this.$emit('loggedIn')
if(this.$route.params.nextUrl != null){
this.$router.push(this.$route.params.nextUrl)
}
else {
if(is_admin== 1){
this.$router.push('admin')
}
else {
this.$router.push('dashboard')
}
}
}
})
.catch(error => {
console.error(error);
this.loading = false
if (error.response.status == 401){
this.error = error.response.data.error
}
});
}
}
},
}
</script>
After add code successfully run your application
Don’t forget to run this flowing command.

After that Received Response from the backend, this loader will automatically hide. So here we will complete a blog post on How To Display A Loader While Sent Request Is In Progress In Vue.
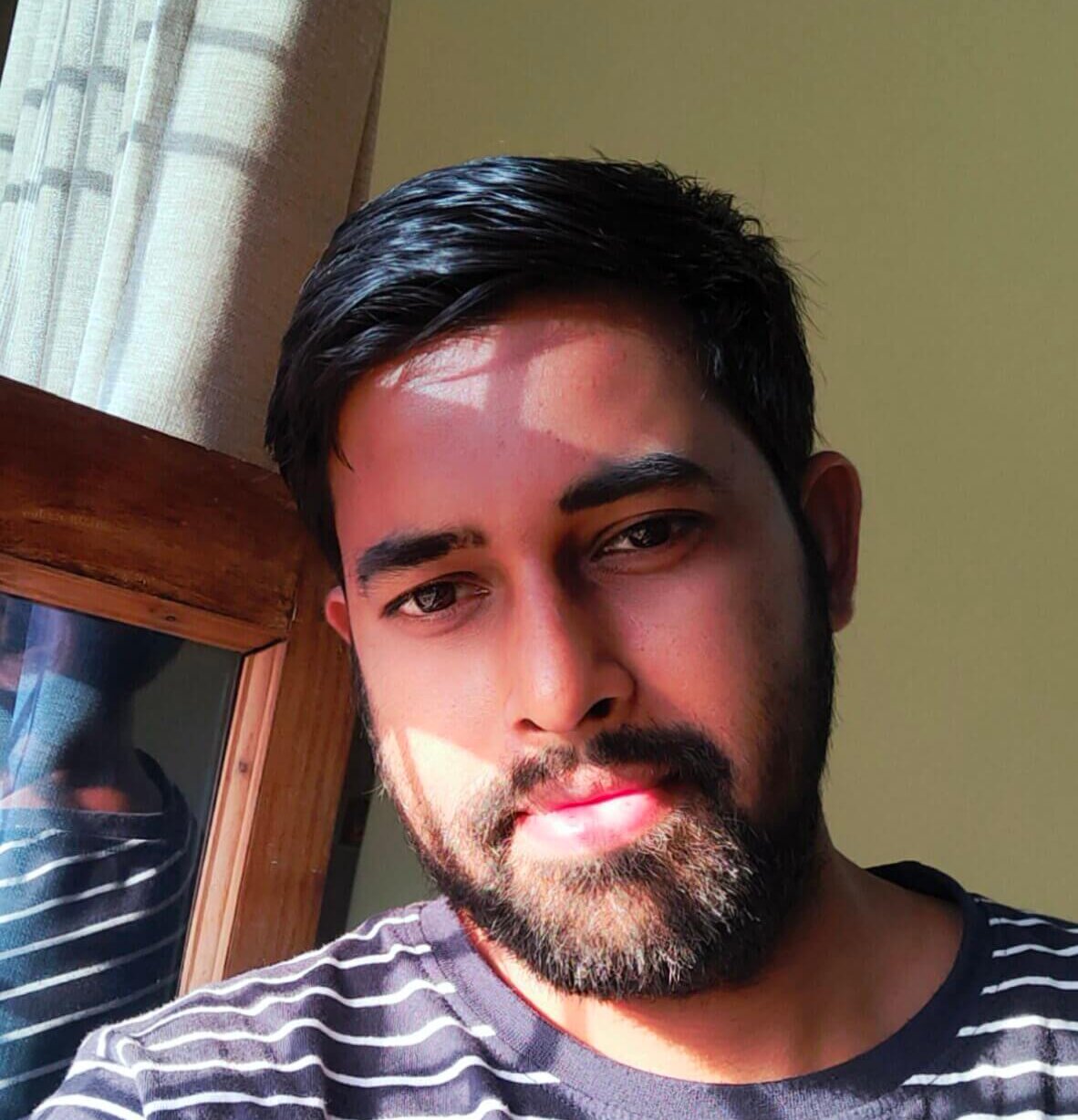
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.