In Python, you can use the re
module to work with regular expressions and check if a string matches a specific regex pattern. Here’s a basic example of how to do this:
import re
# Define the regex pattern
pattern = r'^[a-zA-Z0-9_]+$'
# Input string
input_string = "Hello123"
# Use the re.match() function to check if the string matches the pattern
if re.match(pattern, input_string):
print("String matches the regex pattern.")
else:
print("String does not match the regex pattern.")
In this example, the re.match()
function returns a match object if the input string matches the regex pattern, or None
if it doesn’t. The ^
and $
in the regex pattern represent the start and end of the string, respectively, and [a-zA-Z0-9_]
represents any alphanumeric character or underscore.
You can adjust the pattern
variable to match your specific regex requirements. The re
module provides other functions as well, such as re.search()
and re.findall()
, which you can use depending on your needs.
Keep in mind that regular expressions can vary in complexity, so you might need to adjust the pattern accordingly to match your specific use case.
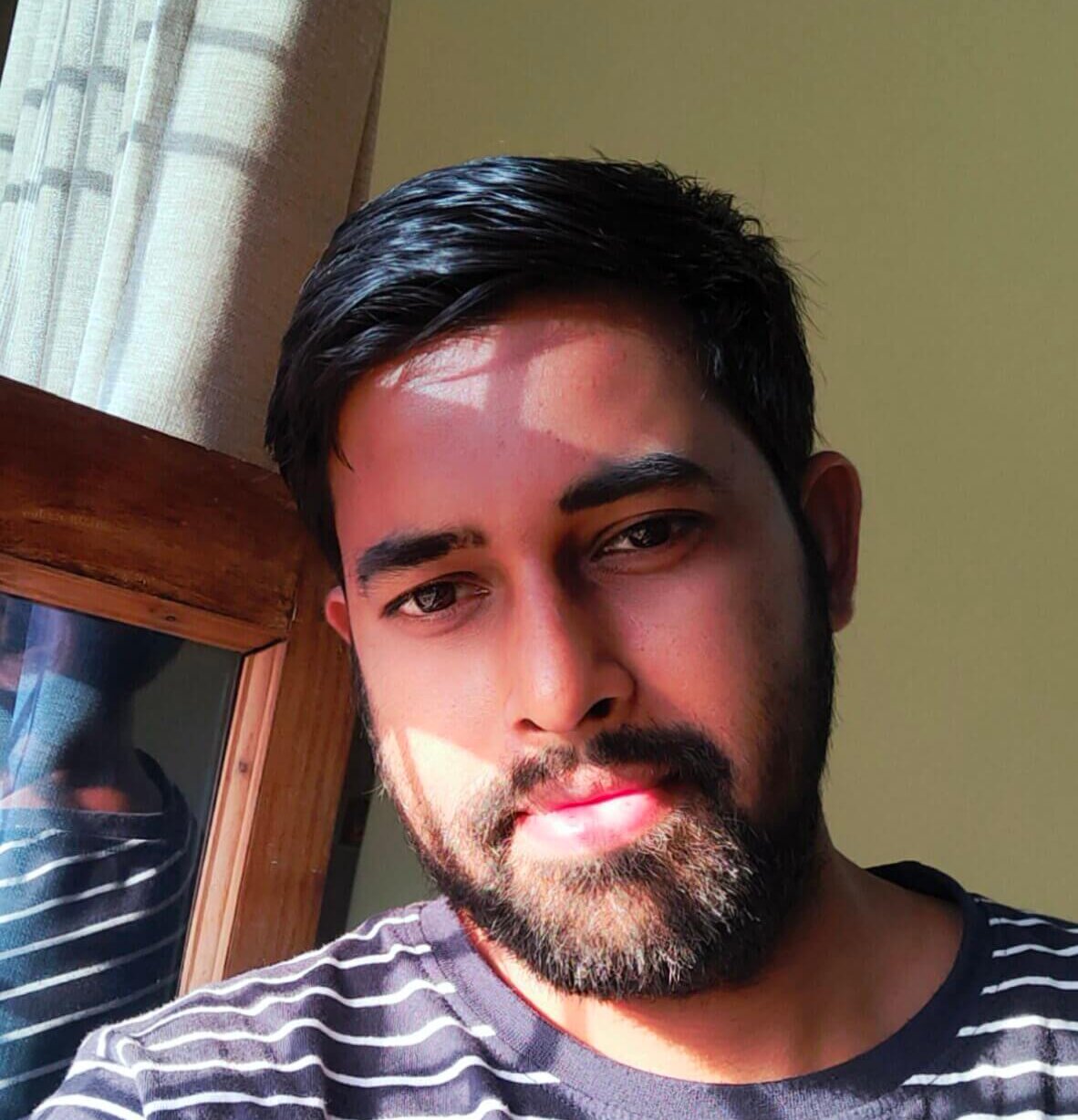
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.