In Python, strings are immutable, which means you cannot directly modify a character at a specific index. However, you can create a new string by replacing the character at the desired index. Here’s an example of how you can replace a character in a string by index:
def replace_char_by_index(string, index, new_char):
if index < 0 or index >= len(string):
# Index out of range
return string
else:
# Create a new string with the replaced character
new_string = string[:index] + new_char + string[index + 1:]
return new_string
# Example usage
original_string = "Hello, world!"
index = 7
replacement_char = "W"
new_string = replace_char_by_index(original_string, index, replacement_char)
print(new_string) # Output: "Hello, World!"
In the replace_char_by_index()
function, we check if the provided index is within the valid range for the given string. If it is, we create a new string by concatenating the substring before the index, the new character, and the substring after the index. The resulting new string will have the desired character replaced at the specified index.
Please note that strings in Python are immutable, so a new string is created every time you perform an operation that modifies the original string.
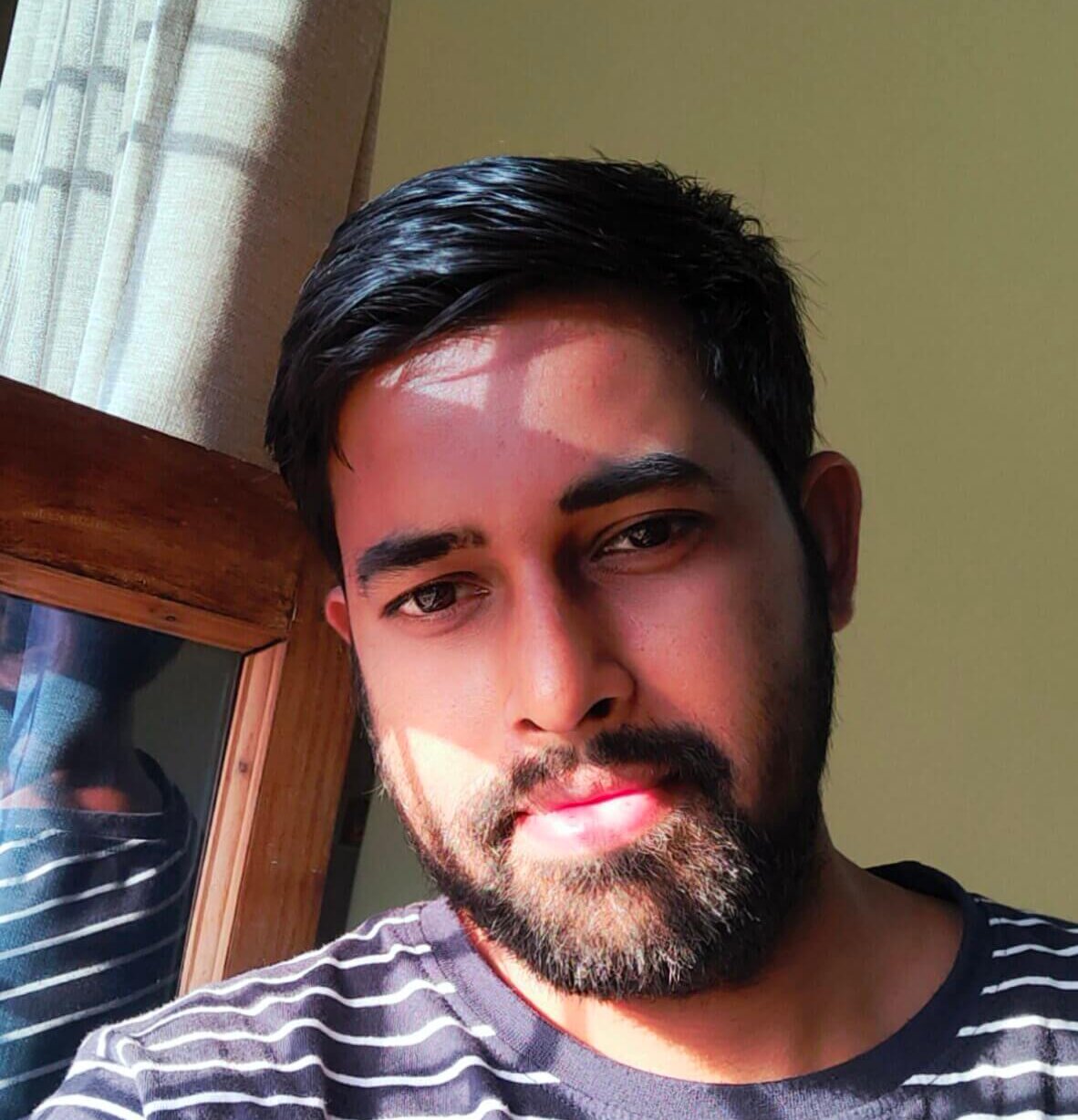
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.