Here’s a code for a random color picker in JavaScript:
HTML code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="src/style.css">
</head>
<body>
<h1 id="header">Hello, Codermen</h1>
<script src="src/script.js"></script>
</body>
</html>
CSS Code
body {
background: transparent; /* Make it white if you need */
color: #fcbe24;
padding: 0 24px;
margin: 0;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif;
}
Javascript Code
function randomColor() {
let color = "#";
let letters = "0123456789ABCDEF";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
let backgroundColor = randomColor();
document.body.style.background = backgroundColor;
This code generates a random hexadecimal color code and sets it as the background color of the webpage.
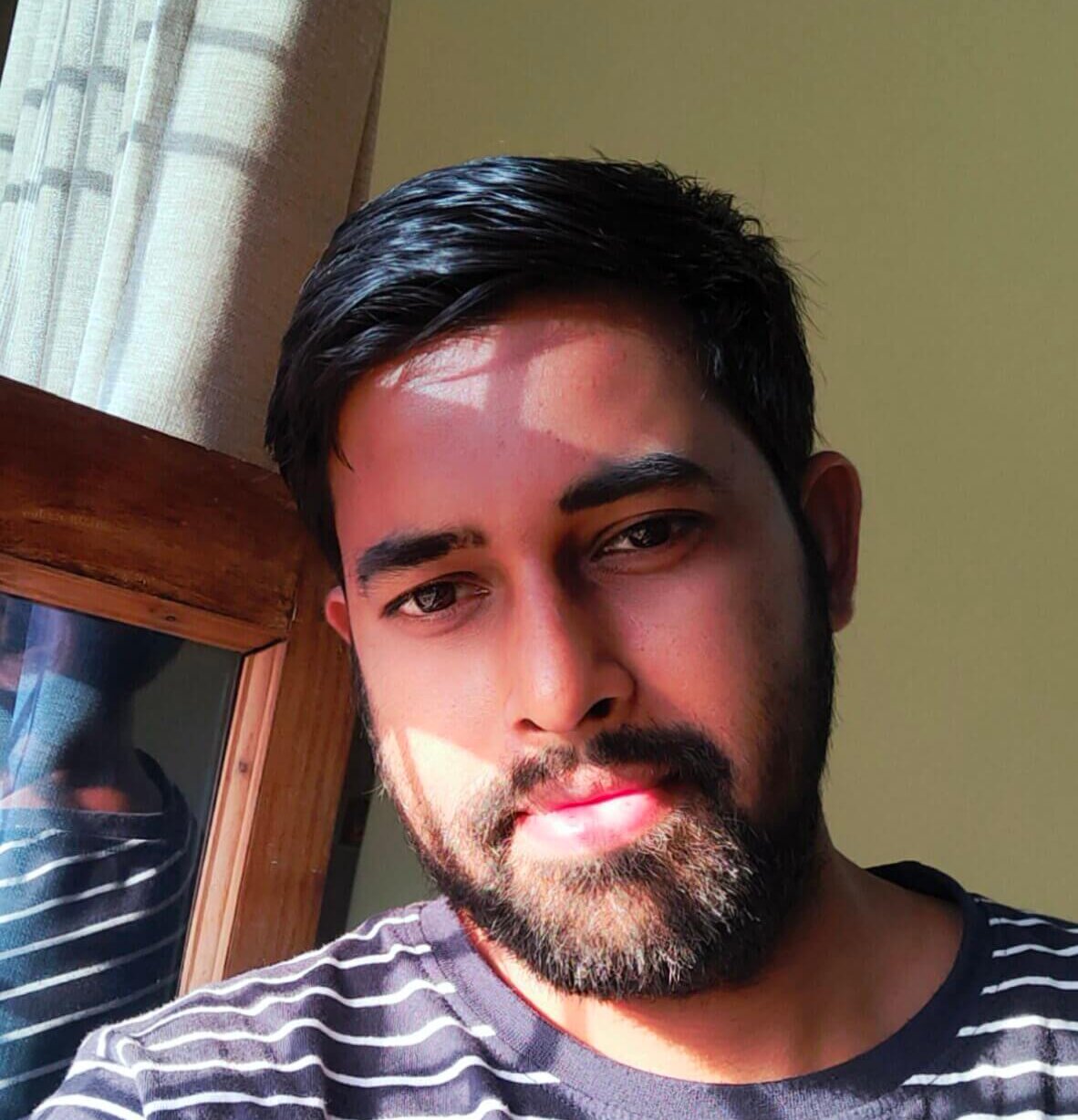
Brijpal Sharma is a web developer with a passion for writing tech tutorials. Learn JavaScript and other web development technology.